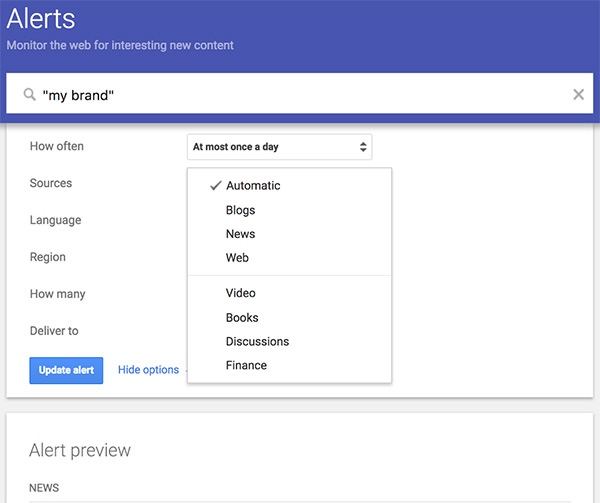
Google Alerts API 1.0
Java Google Alerts API is an easy-to-implement Java library that allows developers to create, delete and update Google alerts within their applications.Overview
Java Google Alerts API provides you with a lightweight library especially designed to help developers create, delete and update Google alerts within their applications. Google alerts will enable users of their software to get the latest relevant Google results based on their preferences. Monitor the Web for interesting new content about your name, brand, competitors, events or any favorite topic. With the help of this component, Java programmers can get Google updates within their software.What Google Alerts give you and what can you expect from your alerts?
Google will start sending email alerts whenever it finds updates matching our keyword on the web. It is one of the most popular services for tracking the Internet. Most professionals use or have used it at some point. Alerts can be used for more than just tracking mentions. You can up your SEO game, identify influencers and enhance your content marketing strategy.You’ll be able to track any keyword on the sources that Google tracks. That means blogs, forums, news sites, and the wider web. It also includes YouTube, since Google owns that platform. Google Alerts will send every mention of these keywords to your email inbox. You can also view them at google.com/alert any time. Google lets you choose the frequency at which you’ll receive them. One option is to get them as they happen. So as soon as your keywords are mentioned online, you’ll be notified (by email). You can also elect to receive all your mentions once per day, or once per week.
This process isn’t real time, and the media monitoring tool doesn’t exactly work like a daily newsletter because it can take Google a few days to index new pages as they’re created. However, for many social media marketing experts or online reputation managers, using Google Alerts is almost as good as getting real-time updates on important issues from all over the web. You can also search all social networks at once for your brand mentions or your hashtags, pulling up a list of relevant posts with details on engagement, reach and sentiment of each.
How to set up Google Alerts
It’s very easy to create useful Google Alerts. Here’s how it works:- Go to google.com/alerts. Make sure you’re logged in with the Google account you want to use.
- Choose your keywords. Try to make them unique. Brand names like Apple and Orange are just going to bring back noise.
- Select the frequency. The choice here is really “real time or when I have time?” If you want to receive notifications in close to real time, select “as it happens.”
- Choose your sources. Google Alerts doesn’t cover social media, but you can choose whether to track news, blogs, videos, or even books.
- Select the language. If you only care about one specific language, now’s your chance to make that choice.
- Choose the region. If your brand is present only in specific countries – or if you’re expanding into new markets – this can be valuable.
- Choose between “only the best” and “everything”. In other words, do you want every mention of your keywords, or will you let Google choose the most important ones?
- Select the email address to deliver to. This will be the one you’re logged into, but you could send your Alerts to an RSS feed if you prefer.
- Hit Create Alert. We’re live!
Java Google Alerts API Usage
// First of all, we have to login. Return true string if login successful else return false GAService service = new GAService("yourGmail", "yourPassword"); service.doLogin(); -- // Get all alerts. List lstAlert = service.getAlerts(); -- // Get alert by delivery. List lstAlert = service.getAlertByDelivery(DeliveryTo.FEED); -- // Get alert by id. Alert alert = service.getAlertById(alertId); -- // Get alert by search query. List lstAlert = service.getAlertsByQuery("Your Query"); -- // Create alert. Return an alert id if created successful else return an error string // Default deliver is email Alert alert = new Alert(); alert.setHowMany(HowMany.ONLY_THE_BEST_RESULTS); alert.setHowOften(HowOften.ONCE_A_DAY); alert.setResultType(ResultType.EVERYTHING); alert.setSearchQuery("Your Query"); alert.setDeliveryTo(DeliverTo.FEED); String id = service.createAlert(alert); -- // Delete an alert. Service.deleteAlert(alertId); -- // Delete list of alerts. List lstAlertId = new ArrayList(); lstAlertId.add(alertId); service.deleteAlert(lstAlertId); -- // Update alert. Return empty string if updated successful else return an error string AlertBean alert = new AlertBean(); alert.setId(editAlertId); alert.setHowMany(HowMany.ONLY_THE_BEST_RESULTS); alert.setHowOften(HowOften.ONCE_A_DAY); alert.setSearchQuery("your new query"); alert.setDeliverTo(DeliverTo.FEED); service.updateAlert(alert);
Python Google Alerts
The google-alerts Python module provides an abstract interface for the Google Alerts service. Google does not provide an official API for this service, so interactions are done through web scripting. Questions, comments or for support needs, please use the issues page on Github. Features- Add new monitors (RSS or Mail)
- Modify existing monitors
- Delete monitors by ID or term
- List all monitors with details
Google Alerts php library
There is no official api for google alerts in PHP, but coders11 php library can create, read or delete programmatically google alerts with a minimum effort. Coders11 has the library already implemented not only in php but in many other programming languages like c# and java, contact coders11 for more information.Google alerts api library Usage Example
//Create a new alert for the query 'php', with default values $ga=new Galerts('user', 'pass'); $alert=$ga->create('php'); var_dump($alert); //print out the alert data //Lists all alerts in the account $lstAlerts=$ga->getList(); var_dump($lstAlerts); //print out the array of alerts //Deletes the first alert of the account $ga->delete($lastAlerts[0]['id']);
Google Alerts Api PHP Specifications
The library simulates the requests to the service through curl in the same way as if you were accessing with a browser. The library is a small class with around 300 lines of code and three public methods with optional parameters. The code is exhaustively tested on many different servers, php versions, languages and locations. Class library instantiation:public function __construct($user, $pass, $timeout=30)
Create google alert api method:
/** * Creates a new alert in the system * @param string $query term to search for * @param string $lang language of the searches ('en', 'ca', 'es', 'fr'…) * @param string $frequency when the alerts are refreshed. Possible values: 'day', 'week', 'happens' * @param string $type type of the returned results. Possible values: 'all', 'news', 'blogs', 'videos', 'forums','books' * @param string $quantity number of results all or just the best. Possible values: 'best', 'all' * @param string $dest destination of the alert. Possible values: 'feed', 'email' * @return array alert data * @throws Exception when the server returns an incorrect response */ public function create($query, $lang='en', $frequency='happens', $type='all', $quantity='best', $dest='feed')
Google alerts api in nodejs
Google Alerts service allows you to create web watchers for specific phrases / words. Unfortunately, official API is not provided by Google. That's why google-alerts-api npm package was made.How Google alerts api in nodejs works? Google-alerts-api npm package sends HTTP GET requests into google.com/alerts and parses it's state object in order to retrieve specific information related to set of already registered alerts. We have two options to authenticate user:
by providing Google account email and password
by providing cookie value, generated earlier
Google Alerts API requires us to specify both deliverTo and deliverToData fields in order to create email based alert.
How to use Google Alerts with Gmail
Setting up a Google Alert for a keyword is easy.-First open your browser and go to google.com/alerts.
-Enter your search query, As you enter your terms, view a preview of the results below. For example, use the search "Top Downloads -shareware" to search for articles where the term "Top Downloads" appears and the shareware is not mentioned. We used the "-" to exclude shareware from the results.
-Choose the result type. Typically "everything" is just fine. Choose Show Options to narrow the alert to a specific source, language, and/or region.
-Choose how often you wish to receive an alert. The "As-it-happens" setting is best if you need immediate notification. For most cases, "once a day" or "once a week" is a good choice.
-Choose whether to receive "only the best results" or "all results". If your search terms are frequently used or return hundreds of results, choose "only the best results".
-How to receive alert: Choose to receive the results by email or an RSS feed. If your keyword requires urgent action, send the results in Gmail. You can also send non-actionable alerts to your RSS reader. Now your alert is set and Google will notify you when the search engine indexes a page that matches the terms you choose.
Most people receive Google Alerts in email. With one or two alerts that track rarely used keywords, you might receive an occasional alert. But try to track several popular topics and alerts can fill your email quickly. That's when Inbox by Gmail comes in handy.
Alerts can flood your inbox, Inbox by Gmail gathers your alerts into a bundle. So create different bundles for different alerts and customize notifications for each bundle. Google now enables Inbox by Gmail by default. To try Inbox, go to inbox.google.com or install the Inbox Android or iOS app on your mobile device.
How to improve your alert results.
You need to improve your search criteria in order to improve your alert results. You need to refine your Alert results by:-If you need to excludes some keyword from the results you need to Place a hyphen in front of a term to exclude, for example use -shareware to exclude all the pages with this search term.
-If you search is a combination of of more that two words try to Add quotes to a specific phrase. For example, "Top Software download" without quotes, will include pages that contains any combination of the three words, top, software, and download.
-Limiting your search to a single domain with the site: term. For example: site:softlookup.com will monitor results only from our website.
What are the benefits of alerts
Google Alerts serve multiple purposes. You can track products, topics, and people. Setup an alert for the name of your organization to track what are people talking about your business and how your campaign are performing and you'll never miss any news you should know about your organization. Having alerts delivered directly to you ensures that you won’t miss any news story for your keyword that don’t show up in Google’s first few pages. It’s a lot quicker and easier to receive an update alert than it is to repeatedly search for a term on Google yourself. You can also start using Google Alerts as a link building tool by setting up alerts for queries related to your product or service, for example Alerts will show you relevant new pages where you can answer somebody’s question, promote your product or brand, and leave a link back to your site.Stay informed with Google Alerts
When you carefully choose your search queries you will get notified when Google finds a match. You can receive alerts containing specific product or service names. Set a Google Alert for "iPhone 11" to learn whenever that term appears online, or to get the latest news on "MAC pro". People who work in marketing, sales can benefit a lot from the use of Google Alerts. It is an easy way to stay up-to-date on what your customers, potential customers are publicly up to online. Google Alerts also helps track much broader topics but it can't find everything, though because google cannot index the whole web. Google Alerts helps you find new blog posts ideas, unique content angles that other content marketers haven’t covered yet. It can also help you find and take advantage of other relevant keywords.How to make your alerts more effective
Here are few things to think about as you start with Google Alerts.- You might think that the more alerts you receive, the better but your inbox will be flooded, and you’ll find yourself deleting them without even looking at them so avoid generic, or common keywords and stick to specific, precise keywords that you know will always be relevant to you.
- To keep your google alerts results precise, you need to setup more than one alert. For example if you need to get notified about issues and problems, you can set individual alerts for each type of problem, and you’ll get specific notifications about each problem you need to solve.
- If you need to get results containing exact phrase, you may need to combine keywords and use speech marks, so your alerts will return results with the exact words.
- Include common misspellings of your search terms. It’s still a good idea to cover all your bases and not excluding results that could add value to you.
- To narrow down your search use filters where possible. Let’s say you’re trying to get traffic to your site, and you’d like to find guest blogging opportunities. Try creating alerts using phrases like “write for us” and then limit your Alerts to blogs.
- Keep your goals in mind as you set up your alerts to get more from your alerts.
-If you use an RSS feed, you can have your alerts sent there instead of to your email. This also prevents your email inbox from getting clogged up.
How to create an alert
Get info about news, products you are interested in, or mentions of your company. You just need to follow the below easy steps: - Use your browse and go to Google Alerts.- In the top box, enter the alert title you want to follow.
- Click Show options if you want to change your Alerts settings.
- Fill in how often you get notifications
- Fill in the types of sites you want view
- Enter the desired language
- Select how many results you want to see
- Select what accounts get the alert
- Now Click Create Alert.
- Now you will receive emails whenever Google finds results matching your search criteria.
How to Edit an alert
- To Edit an alert you need to go to Google Alerts.- Now Click Edit.
- You can now Make the changes needed.
- Then Click Update Alert.
- To change how you get alerts, click Settings Settings and then check the options you want and click Save.
How to Delete an alert
- To delete an alert you need to go to Google Alerts.- Navigate to the Alerts you want to delete and click on Delete.
- You can also click on Unsubscribe at the bottom of an alert email to delete an alert.
How to Check what account you’re logged onto
- You need to go to Google Alerts.- Now, Check the current account in the Google Bar.
- Select your account by clicking on your profile image.
- If you are not on the correct account then click on Sign Out next to the profile image.
- Click on Sign in with another account.
I am not getting any new alerts, Why?
- First you need to check if your inbox isn't full- Check if your alerts have been disabled
- Check if you Google Alert emails are going to your spam folder
- If so add googlealerts-noreply@google.com to your contacts
Summary
Google Alerts is so important for marketers or social media managers because it keep them aware of what people are saying about your business online. So start tracking your most important names and keywords today and you might be surprised at what you results you will find!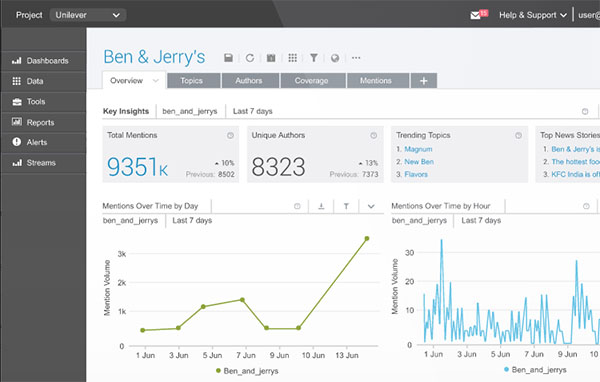
Google Alerts Alternatives
If you want to boost your social listening and monitoring process, you may need to check some advanced tools other than Google Alerts that track mentions across the web, social media, blogs, etc. We will list some of these tools with their specific benefits.Talkwalker Alerts is Google Alert alternative that exists on the market. It is a powerful social media monitoring and analytics tool that monitors most of the web, including blogs and forums. Talkwalker Alerts is absolutely free, and very easy to use. You choose a keyword to monitor, leave your email, and you'll get listings of relevant mentions delivered to your inbox.
What can I do with Talkwalker Alerts?
Talkwalker Alerts can be used in multiple ways.
1- If you’re a job seeker, our free marketing tool is your best friend. You can use Talkwalker Alerts to find jobs in two ways:
Set up an alert for your dream companies. Every time a new job is posted on their website, you’ll receive alerts.
Filter jobs by the position you’re looking at and the country. For instance, an alert query such as "marketing manager" AND source country: FR, will show you all results for marketing manager positions in France.
2- SEO and outreach
If you’re looking to enhance your website’s SEO and have a massive list of websites to reach out to, Talkwalker Alerts will help. Since we deliver results from the internet to your inbox every day, you’ll have a list of websites that mentioned your brand or your keyword and you can reach out to them and ask them for a backlink, instead of performing a Google search each day or logging into another tool. Additionally, since we bring you the tweets with the most engagement, you’ll also have a bunch of influencers to reach out to for collaboration and partnership opportunities.
3- Brand mentions
If you’re looking to simply track your brand or keyword online, Talkwalker Alerts will help you bring all your brand mentions from across the internet to your inbox. It’s pretty useful for daily stand-up meetings or quick reports on your online brand health.With the addition of Twitter results, it can also be used as a very basic CRM tool.
Brandwatch is a powerful, enterprise-level tool that monitors every social network you can think of. It monitors all review sites, articles, comment sections, and anything else you can find on the web. The coverage is detailed, complete, and is updated all the time. Unlike most tools, Brandwatch also provides image recognition capacity. This enables you to find images that contain your Company’s logo.
Awario is one of the newest social listening tools on the market. Awario monitors all the major social media networks, blogs, forums, news sites, and the rest of the web in real time. The tool also enables you to reply to mentions straight from your app, and from multiple social media accounts.
Mention is one of the oldest social media monitoring tools. The tool monitors all the major social media networks, blogs, news, forums, and the web in real time. Mention can also highlight brand-relevant social media influencers, and it has tools for analysis and sentiment. Mention is made for social media marketing teams.
Brand24 monitors all the major social media platforms, news, blogs, and the web. It analyzes mention growth, reach, sentiment, and it can also find relevant social media influencers. The mentions are updated every 12 hours in the Personal plan, and every hour in the Premium plan, and in real time for the users of the most expensive plan.
Conclusion
To conclude Google Alerts API works on Windows operating system(s) and can be easily downloaded using the below download link according to Freeware license. Google Alerts API download file is only 14 KB in size.Google Alerts API was filed under the General category and was reviewed in softlookup.com and receive 5/5 Score.
Google Alerts API has been tested by our team against viruses, spyware, adware, trojan, backdoors and was found to be 100% clean. We will recheck Google Alerts API when updated to assure that it remains clean.
Google Alerts API user Review
Please review Google Alerts API application and submit your comments below. We will collect all comments in an effort to determine whether the Google Alerts API software is reliable, perform as expected and deliver the promised features and functionalities.Popularity 10/10 - Downloads - 2651 - Score - 5/5
Softlookup.com 2023 - Privacy Policy
Category: | General |
Publisher: | Ha Nguyen |
Last Updated: | 19/11/2023 |
Requirements: | Not specified |
License: | Freeware |
Operating system: | Windows |
Hits: | 10698 |
File size: | 14 KB |
Price: | Not specified |
Leave A comment | |
Name: * |
E-Mail: * |
Comment: * |